Map Utilities
defaultValues
info
The bounds
property will overwrite all the other properties
The Map Render component needs default values to be rendered on screen.
You can set those values:
center
: If you're not passing any default value, the value ofsetMapCenter
will be used, and if there's still no value there a{lat:0, lng:0}
will be used as throwback.zoom
: If you're not passing any default value, the value ofsetMapZoom
will be used, and if there's still no value there a0
will be used as throwback.bounds
: If you're passing no values, the bounds will be ignored.
<MapRender
defaultValues={{
center: { lat: 9, lng: 25 },
zoom: 3,
bounds: [
{ lat: 30, lng: 45 },
{ lat: 12, lng: 32 },
],
}}
/>
getMapInfo
You can use getMapInfo
as prop with the Map Render component
<MapRender getMapInfo={{ dispatch: setMapInfo }} />
the object's type is GetMapInfo
:
type GetMapInfo = {
dispatch: React.Dispatch<React.SetStateAction<any>>;
debounceTime?: number;
};
Everytime one of the properties change (dragging map, zooming, drawing...), you will receive informations about the current state of these properties in the dispatch
function:
- zoom: a number value
- bounds: an array value
- shapeCoordinates: If you're using activateControls the function will also send information about the shapes in the
shapeCoordinates
's value. - savePolygon:
true
if the user clicks on PopUp to save current polygon generated by activateSave
The debounceTime
is the time Crono
will take to give you informations after the user has finished interacting with the map (a default value has been set)
setMapCenter
info
You can't use this function if you're passing bounds to the map
You can set the central coordinate of the map with setMapCenter
as prop with the Map Render component
<MapRender setMapCenter={{ lat: 0, lng: 0 }} />
The object's type is LngLatLike
setMapZoom
info
You can't use this function if you're passing bounds to the map
You can set the zoom of the map with setMapZoom
as prop with the Map Render component
<MapRender setMapZoom={10} />
The object's type is number
setMapBounds
info
Will override all other geographics value and setters
You can set the bounts of the map with setMapBounds
as prop with the Map Render component
<MapRender
setMapBounds={[
{ lat: 0, lng: 0 },
{ lat: 0, lng: 0 },
]}
/>
The object's type is number
isLoading
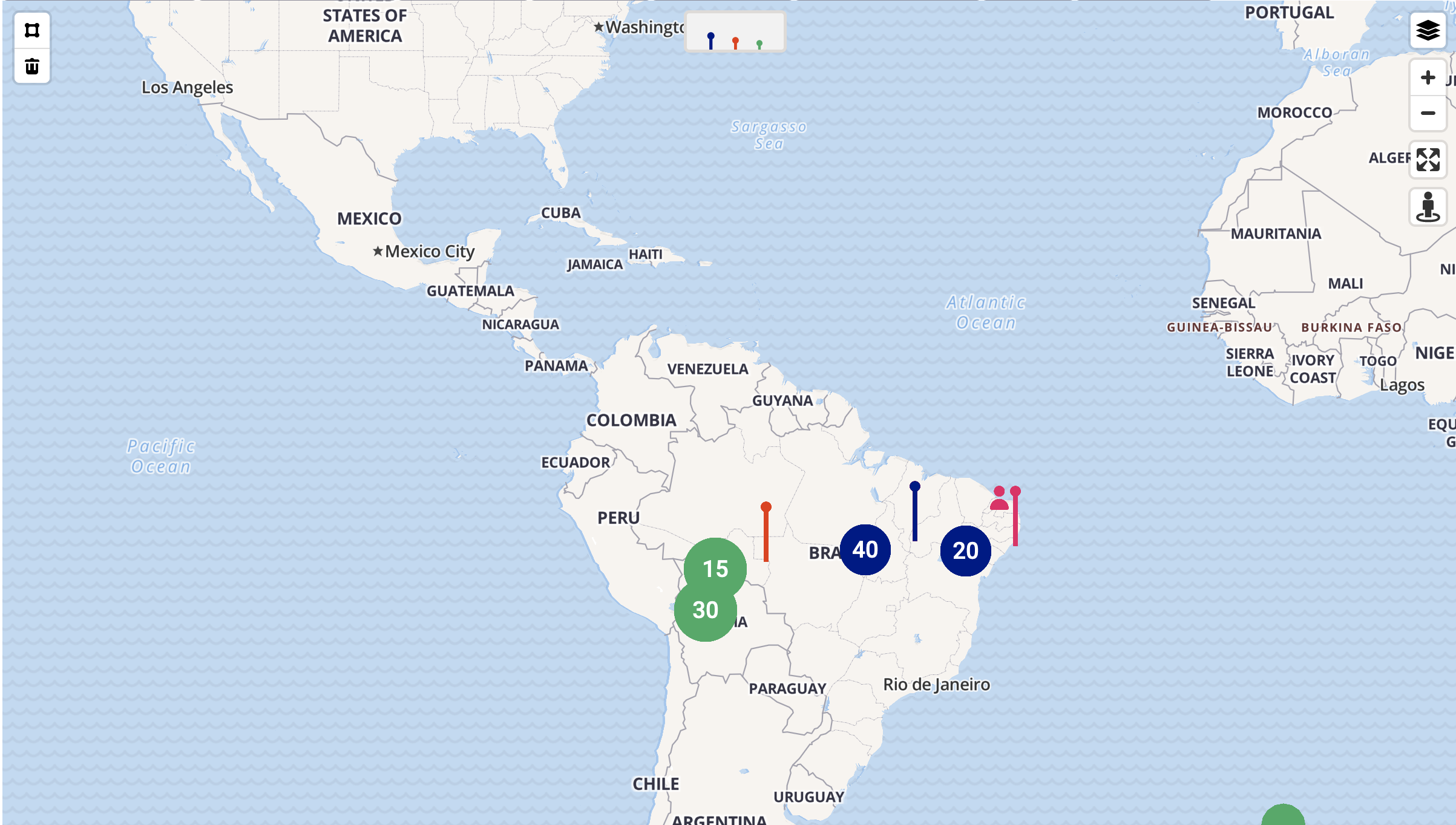
You can toggle a loading visualization on the map by setting isLoading
as true
on the Map Render component
<MapRender isLoading={true} />
The object's type is boolean
onToggleCluster
You can get back informations about current toggling.
The onToggleCluster
gives you back if you're showing Heatmap or Pointer Clusters
<MapRender
heatClusterToggle={{
activateControl: true,
onToggleCluster: (data) => getCurrentGeoJsonOnToggleCluster(data),
}}
/>
The object's type is OnToggleCluster